Blog
Understanding the BLoC Pattern in Flutter: A Complete Guide
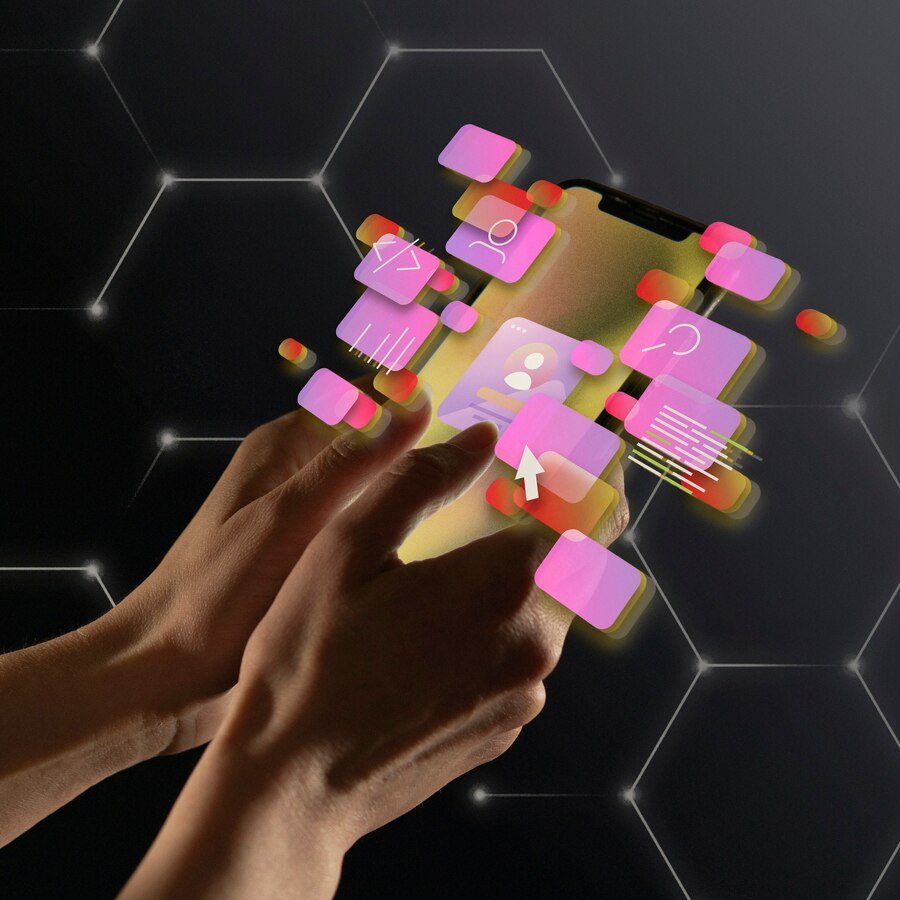
Flutter is a powerful framework for building cross-platform mobile applications. As apps grow in complexity, state management becomes a crucial factor in ensuring efficiency and maintainability. One of the most popular state management solutions in Flutter is the BLoC (Business Logic Component) pattern. BLoC helps separate UI from business logic, making apps scalable, testable, and reusable. In this guide, we will explore what BLoC is, why it is useful, and how to implement it in a Flutter app.
What is the BLoC Pattern?
BLoC is a design pattern that separates business logic from the UI, ensuring that an app remains clean and organized. It uses Streams and Sinks to manage state in a reactive way, ensuring efficient communication between UI components and the logic layer.
Key Components of BLoC
- Events – Actions triggered by the user or system.
- Bloc (Business Logic Component) – Handles events, processes logic, and outputs states.
- States – UI representations of different application conditions.
- Stream – A pipeline for sending and listening to data asynchronously.
Why Use the BLoC Pattern?
Separation of Concerns – Keeps UI code separate from business logic.
Improved Testability – BLoC can be tested independently without relying on UI components.
Scalability – Suitable for large applications with multiple screens and complex state transitions.
Reactive Programming – Uses Streams to handle events efficiently.
Setting Up BLoC in a Flutter Project
To use the BLoC pattern in Flutter, follow these steps:
Step 1: Add Dependencies
Install the flutter_bloc package by adding it to your pubspec.yaml file:
yaml
CopyEdit
dependencies:
flutter_bloc: ^8.1.2
Run the following command to fetch dependencies:
sh
CopyEdit
flutter pub get
Step 2: Create the Event
Define events that trigger state changes.
Create a file counter_event.dart:
dart
CopyEdit
abstract class CounterEvent {}
class Increment extends CounterEvent {}
class Decrement extends CounterEvent {}
Step 3: Create the State
Define the state of the app.
Create a file counter_state.dart:
dart
CopyEdit
class CounterState {
final int counterValue;
CounterState(this.counterValue);
}
Step 4: Create the BLoC
The BLoC class handles events and produces new states.
Create a file counter_bloc.dart:
dart
CopyEdit
import ‘package:flutter_bloc/flutter_bloc.dart’;
import ‘counter_event.dart’;
import ‘counter_state.dart’;
class CounterBloc extends Bloc<CounterEvent, CounterState> {
CounterBloc() : super(CounterState(0)) {
on<Increment>((event, emit) => emit(CounterState(state.counterValue + 1)));
on<Decrement>((event, emit) => emit(CounterState(state.counterValue – 1)));
}
}
Step 5: Integrate BLoC in the UI
Use BlocProvider to provide the BLoC to the widget tree.
Modify main.dart:
dart
CopyEdit
import ‘package:flutter/material.dart’;
import ‘package:flutter_bloc/flutter_bloc.dart’;
import ‘counter_bloc.dart’;
import ‘counter_event.dart’;
import ‘counter_state.dart’;
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return BlocProvider(
create: (context) => CounterBloc(),
child: MaterialApp(
home: CounterScreen(),
),
);
}
}
class CounterScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text(‘BLoC Counter’)),
body: Center(
child: BlocBuilder<CounterBloc, CounterState>(
builder: (context, state) {
return Text(
‘Counter: ${state.counterValue}’,
style: TextStyle(fontSize: 24),
);
},
),
),
floatingActionButton: Row(
mainAxisAlignment: MainAxisAlignment.end,
children: [
FloatingActionButton(
onPressed: () =>
context.read<CounterBloc>().add(Increment()),
child: Icon(Icons.add),
),
SizedBox(width: 10),
FloatingActionButton(
onPressed: () =>
context.read<CounterBloc>().add(Decrement()),
child: Icon(Icons.remove),
),
],
),
);
}
}
Understanding the Code

- BlocProvider provides the BLoC instance to widgets.
- BlocBuilder listens for state changes and rebuilds the UI.
- context.read<CounterBloc>().add(Increment()) sends an event to update the state.
- State updates trigger UI changes automatically.
Best Practices for Using BLoC
Keep events and states simple – Avoid unnecessary complexity.
Use BlocProvider at the top level – Ensures the BLoC is available throughout the widget tree.
Utilize BlocListener – When performing side effects like navigation or showing snackbars.
Write Unit Tests – Test business logic separately from UI.
Conclusion
The BLoC pattern is a structured and scalable approach to state management in Flutter. By separating UI from business logic, BLoC ensures maintainability, reusability, and testability. While it may require a bit of a learning curve, the long-term benefits make it a preferred choice for handling complex states in Flutter applications.
FAQs
What is the BLoC pattern in Flutter?
BLoC (Business Logic Component) is a state management pattern that separates UI from business logic using streams and events.
How does BLoC differ from Provider?
Provider is simpler and lightweight, whereas BLoC is more structured and scalable, making it better for complex applications.
Is BLoC suitable for small apps?
BLoC is powerful, but for very small apps, simpler state management solutions like Provider or setState may be sufficient.
Does BLoC work with Flutter Web and Desktop?
Yes! BLoC is platform-agnostic and works across mobile, web, and desktop applications.
What are alternatives to BLoC in Flutter?
Other state management options include Provider, Riverpod, Redux, GetX, and MobX.
-
Business1 year ago
Sepatuindonesia.com | Best Online Store in Indonesia
-
Tech5 months ago
How to Use a Temporary Number for WhatsApp
-
Technology9 months ago
Top High Paying Affiliate Programs
-
Tech1 month ago
Understanding thejavasea.me Leaks Aio-TLP: A Comprehensive Guide
-
Social Media8 months ago
The Best Methods to Download TikTok Videos Using SnapTik
-
Technology5 months ago
Leverage Background Removal Tools to Create Eye-catching Videos
-
Instagram3 years ago
Free Instagram Follower Without Login
-
Instagram3 years ago
Free Instagram Auto Follower Without Login